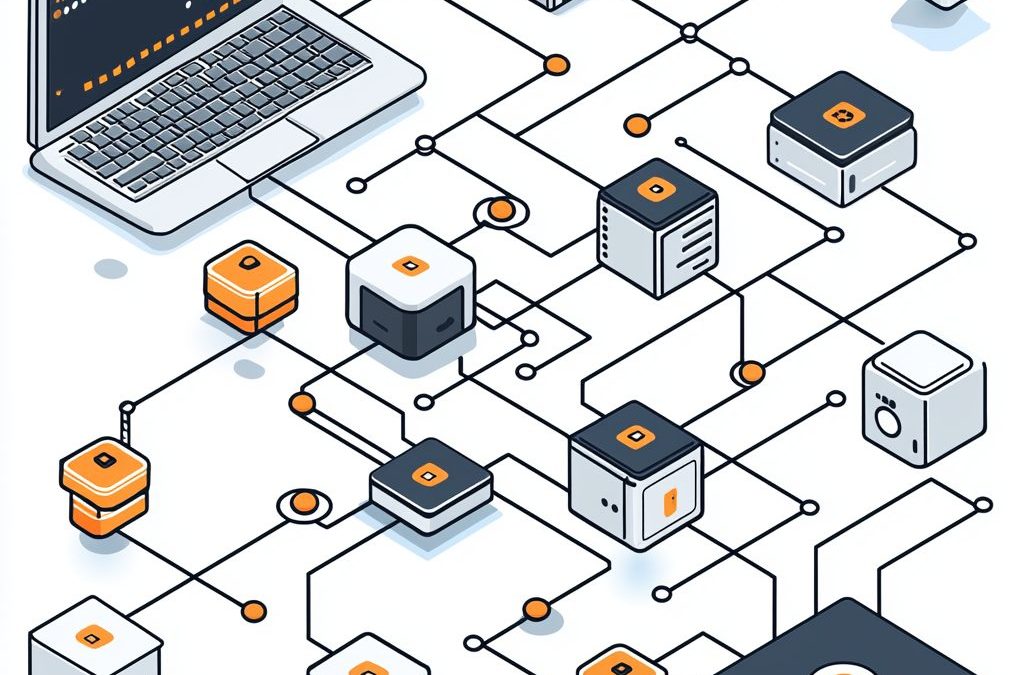
Understanding Linux Polling: How It Works
Polling in Linux is a fundamental technique used in system programming to monitor multiple file descriptors to see if any of them is ready for some class of I/O operation (e.g., reading or writing).
In environments where multiple I/O sources need to be managed, such as network servers, user interfaces, or event-driven systems, polling allows for efficient resource utilization by checking the status of multiple resources without blocking on any single one.
Polling forms the backbone of many I/O multiplexing mechanisms, which are essential for building scalable and responsive applications in Linux.
The core idea behind polling is to enable a process to manage multiple input/output sources efficiently without dedicating a separate thread or process to each one. I will explain how polling works in Linux, covering its underlying concepts, various polling mechanisms available, and practical examples to illustrate their use.
The Concept of Polling
What is Polling?
Polling is a technique where a process or a thread repeatedly checks the status of an I/O operation, typically in a non-blocking manner. In the context of Linux and Unix-like operating systems, polling refers to monitoring multiple file descriptors to determine if they are ready for I/O operations.
Why Polling?
In a typical I/O operation, a process might block while waiting for data to become available. This blocking can be inefficient in scenarios where a process needs to handle multiple I/O sources, such as in network servers that handle many client connections.
Polling allows the process to monitor multiple file descriptors simultaneously, and respond only when one or more of them are ready for I/O operations, thereby improving the efficiency of the application.
Synchronous vs. Asynchronous I/O
Polling is often discussed in the context of synchronous I/O, where the process actively waits for I/O events to happen. This is in contrast to asynchronous I/O, where the kernel notifies the process when an I/O event has occurred, typically via signals or callbacks.
Polling Mechanisms in Linux
Linux provides several mechanisms to implement polling, each with its own strengths and weaknesses. The choice of mechanism depends on the specific requirements of the application, such as the number of file descriptors being monitored, performance considerations, and portability across different Unix-like systems.
poll()
The poll()
system call is one of the most commonly used polling mechanisms in Linux. It allows a process to monitor multiple file descriptors to see if any of them are ready for I/O. The poll()
function works by taking an array of struct pollfd
and a timeout value, then blocking until one or more file descriptors are ready or the timeout expires.
How poll()
Works
Here is a simple example of how poll()
can be used:
#include <poll.h>
#include <unistd.h>
#include <stdio.h>
int main() {
struct pollfd fds[2];
int ret;
// Monitor stdin (file descriptor 0) for input
fds[0].fd = 0; // stdin
fds[0].events = POLLIN;
// Monitor a file descriptor for output readiness
fds[1].fd = 1; // stdout
fds[1].events = POLLOUT;
// Wait for an event on one of the file descriptors
ret = poll(fds, 2, -1);
if (ret > 0) {
if (fds[0].revents & POLLIN) {
printf("Data is available to read on stdin.\n");
}
if (fds[1].revents & POLLOUT) {
printf("File descriptor 1 is ready for writing.\n");
}
} else {
printf("Poll failed or timed out.\n");
}
return 0;
}
In this example, poll()
monitors the standard input (stdin) and output (stdout) file descriptors for readiness. The function will block until there is data to read from stdin or stdout is ready to accept data.
poll()
Structure and Usage
struct pollfd
: Each file descriptor to be monitored is represented by astruct pollfd
, which includes:fd
: The file descriptor to monitor.events
: The events of interest (e.g.,POLLIN
,POLLOUT
, etc.).revents
: The events that actually occurred, set by the kernel.
- Timeout:
poll()
accepts a timeout parameter, which specifies how long the function should block:-1
: Block indefinitely until an event occurs.0
: Return immediately, even if no events are ready (non-blocking).> 0
: Block for the specified number of milliseconds.
- Return Value: The return value of
poll()
indicates the number of file descriptors that have events ready. A negative return value indicates an error.
select()
select()
is another system call used for monitoring multiple file descriptors. It predates poll()
and is available on most Unix-like systems, making it more portable but less powerful in some respects.
How select()
Works
select()
allows a process to monitor multiple file descriptors by specifying them in three separate sets: one for reading, one for writing, and one for exceptional conditions.
Here’s an example of select()
:
#include <sys/select.h>
#include <unistd.h>
#include <stdio.h>
int main() {
fd_set read_fds;
fd_set write_fds;
struct timeval timeout;
int ret;
// Initialize file descriptor sets
FD_ZERO(&read_fds);
FD_ZERO(&write_fds);
// Monitor stdin for input
FD_SET(0, &read_fds);
// Monitor stdout for output readiness
FD_SET(1, &write_fds);
// Set a timeout of 5 seconds
timeout.tv_sec = 5;
timeout.tv_usec = 0;
// Wait for an event on one of the file descriptors
ret = select(2, &read_fds, &write_fds, NULL, &timeout);
if (ret > 0) {
if (FD_ISSET(0, &read_fds)) {
printf("Data is available to read on stdin.\n");
}
if (FD_ISSET(1, &write_fds)) {
printf("File descriptor 1 is ready for writing.\n");
}
} else if (ret == 0) {
printf("Select timed out.\n");
} else {
printf("Select failed.\n");
}
return 0;
}
In this example, select()
monitors stdin and stdout for readiness. The function blocks until either file descriptor is ready or the timeout expires.
select()
Structure and Usage
- File Descriptor Sets:
select()
usesfd_set
structures to represent sets of file descriptors to monitor:FD_SET(fd, &set)
: Adds a file descriptor to the set.FD_CLR(fd, &set)
: Removes a file descriptor from the set.FD_ISSET(fd, &set)
: Checks if a file descriptor is set.
- Timeout: Similar to
poll()
,select()
accepts a timeout parameter in the form of astruct timeval
, which specifies the number of seconds and microseconds to wait. - Return Value: The return value indicates the number of file descriptors ready for I/O. A return value of 0 indicates a timeout, and a negative value indicates an error.
epoll()
epoll()
is a more advanced and scalable polling mechanism introduced in Linux 2.5. It is specifically designed to efficiently handle large numbers of file descriptors, making it suitable for high-performance servers.
How epoll()
Works
epoll()
works differently from poll()
and select()
in that it uses a kernel-managed event queue. File descriptors are registered with the epoll
instance, and the kernel will notify the process when any of them are ready.
Here’s a basic example of using epoll()
:
#include <sys/epoll.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
int epoll_fd = epoll_create1(0);
if (epoll_fd == -1) {
perror("epoll_create1");
exit(EXIT_FAILURE);
}
struct epoll_event event;
struct epoll_event events[10];
event.events = EPOLLIN;
event.data.fd = 0; // stdin
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, 0, &event) == -1) {
perror("epoll_ctl");
exit(EXIT_FAILURE);
}
int n = epoll_wait(epoll_fd, events, 10, -1);
for (int i = 0; i < n; i++) {
if (events[i].data.fd == 0) {
printf("Data is available to read on stdin.\n");
}
}
close(epoll_fd);
return 0;
}
In this example, epoll()
monitors stdin for input. The epoll_wait()
function blocks until an event occurs on the file descriptor.
epoll()
Structure and Usage
epoll_create1()
: Creates an epoll instance, returning a file descriptor for the event queue.epoll_ctl()
: Manages file descriptors associated with the epoll instance:EPOLL_CTL_ADD
: Add a file descriptor to the epoll instance.EPOLL_CTL_MOD
: Modify an existing file descriptor in the epoll instance.EPOLL_CTL_DEL
: Remove a file descriptor from the epoll instance.
epoll_wait()
: Waits for events on the file descriptors registered with the epoll instance. It returns the number of file descriptors that are ready.- Edge-Triggered vs. Level-Triggered:
epoll
supports two modes:- Level-Triggered (default): Similar to
poll()
, where readiness is checked continuously. - Edge-Triggered: Provides notifications only when the state of a file descriptor changes, which can reduce the number of events handled but requires careful management.
- Level-Triggered (default): Similar to
ppoll()
and pselect()
ppoll()
and pselect()
are variations of poll()
and select()
that offer additional functionality, specifically the ability to handle signals in a race-free manner. These functions allow the program to block on I/O and handle signals atomically, which can be useful in complex applications where signal handling is necessary.
ppoll()
ppoll()
is similar to poll()
but with an additional feature: it allows the program to specify a signal mask, which is atomically set during the execution of ppoll()
. This prevents race conditions where a signal could arrive and be missed while the process is transitioning into a blocking state.
pselect()
pselect()
is the counterpart to select()
, offering the same functionality with the added ability to set a signal mask atomically. It is useful in scenarios where signal handling and file descriptor monitoring need to be tightly integrated.
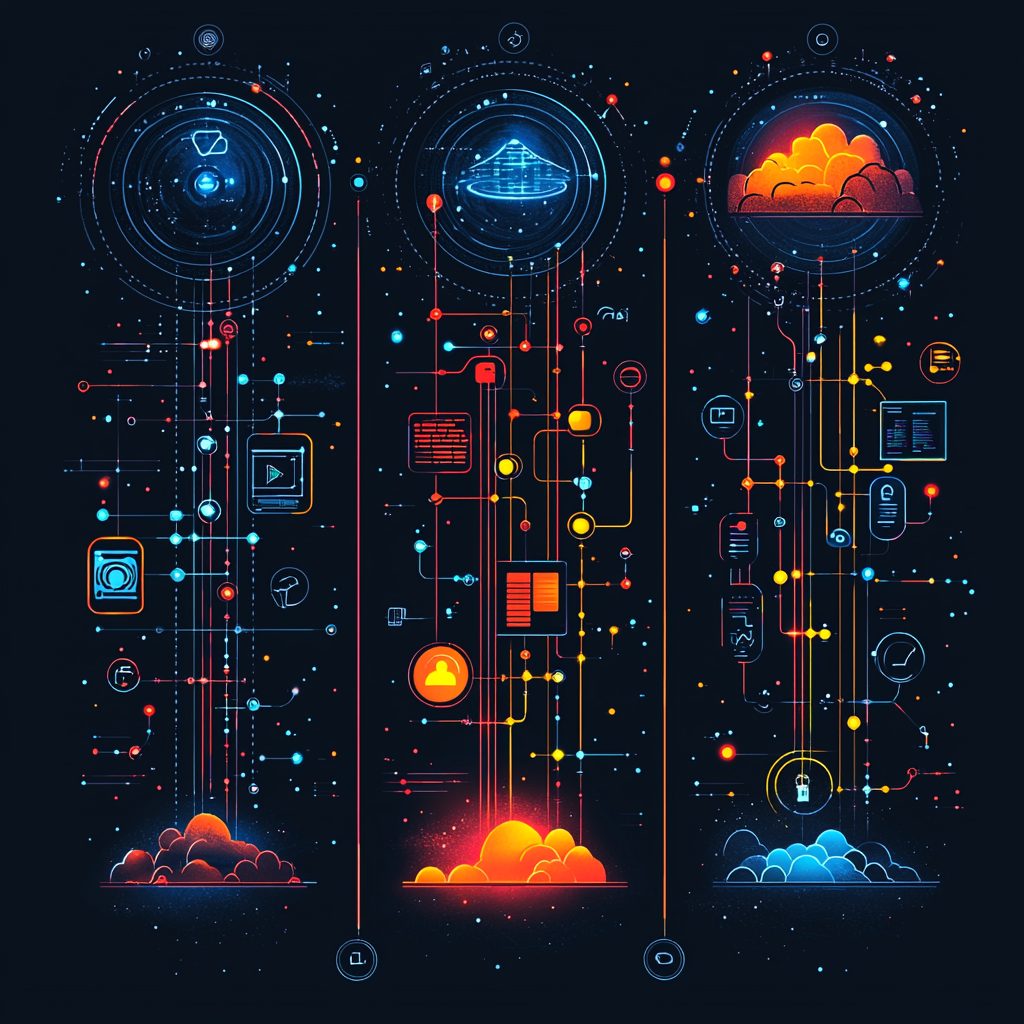
Advantages and Disadvantages of Polling Mechanisms
Each polling mechanism in Linux has its own set of advantages and disadvantages, making it suitable for different types of applications.
poll()
- Advantages:
- Simpler to use compared to
epoll
. - Supported across many Unix-like systems, making it more portable.
- Simpler to use compared to
- Disadvantages:
- Less efficient for a large number of file descriptors because it scans the entire list of descriptors each time.
- The entire list of file descriptors must be passed to the kernel on each call, which can be expensive in terms of CPU usage.
select()
- Advantages:
- High portability across different Unix-like systems.
- Simple and well-understood by many developers.
- Disadvantages:
- Limited by the maximum number of file descriptors (usually 1024 on many systems).
- Inefficient for large numbers of file descriptors, as it requires scanning the entire set on each call.
epoll()
- Advantages:
- Highly scalable, capable of handling thousands of file descriptors efficiently.
- Uses edge-triggered notifications, which can reduce the number of events processed.
- Maintains a kernel-managed event list, reducing the overhead associated with passing file descriptors between user space and the kernel.
- Disadvantages:
- More complex API compared to
poll()
andselect()
. - Not available on non-Linux systems, limiting portability.
- More complex API compared to
ppoll()
and pselect()
- Advantages:
- Enhanced control over signal handling, making them suitable for applications that need to handle signals and I/O simultaneously.
- Helps prevent race conditions related to signals and blocking I/O.
- Disadvantages:
- Slightly more complex to use due to the additional signal mask parameters.
- Limited use cases compared to the more general
poll()
andselect()
.
Practical Considerations
When choosing a polling mechanism, several factors should be considered, including the scale of the application, portability requirements, and specific performance needs.
Scalability
For applications that need to handle a large number of file descriptors, such as high-performance servers, epoll()
is usually the best choice due to its scalability. It is designed to handle thousands of file descriptors with minimal overhead.
Portability
If the application needs to be portable across different Unix-like systems, select()
or poll()
may be more appropriate, as these are supported on a wider range of platforms.
Simplicity
For simpler applications or when dealing with a small number of file descriptors, select()
or poll()
might be preferred due to their simpler APIs and easier integration into existing codebases.
Signal Handling
In cases where signal handling is critical, ppoll()
or pselect()
should be used to avoid race conditions between signals and I/O operations.
Advanced Topics in Polling
Combining Polling with Asynchronous I/O
While polling is a powerful technique, it can be combined with asynchronous I/O mechanisms to create even more efficient and responsive applications. For example, a program might use epoll()
to monitor a large number of network sockets while also using asynchronous I/O for disk operations.
Non-blocking I/O
In many cases, polling is used in conjunction with non-blocking I/O. When a file descriptor is set to non-blocking mode, operations like read()
or write()
will return immediately if they cannot proceed, instead of blocking. This allows the program to continue processing other tasks and use polling to check when the operation can be retried.
Handling Large Numbers of Events
When dealing with a very high number of events, such as in network servers handling thousands of connections, additional strategies can be employed to manage load, such as using worker threads or processes to handle groups of file descriptors, or employing techniques like load balancing across multiple event loops.
Conclusion
Polling is an essential technique in Linux system programming, enabling efficient I/O multiplexing for applications that need to handle multiple input/output sources simultaneously. While select()
and poll()
provide basic polling capabilities, epoll()
offers advanced features and scalability suitable for high-performance applications.
The choice of polling mechanism depends on the specific needs of the application, including considerations of performance, portability, and complexity.
By understanding the strengths and weaknesses of each polling mechanism, developers can select the appropriate tool to build efficient, responsive, and scalable applications in Linux.
FAQ
Q: What exactly is polling in the Linux operating system?
A: Polling in Linux helps manage how data is read and written. It watches many files at once for activities. This makes sure operations don’t block each other.
Q: How is polling different from other methods like select and epoll?
A: Polling, select, and epoll notify about I/O events differently. Polling is simple for non-blocking tasks. Select, though older, struggles with lots of files. Epoll works best when you have many activities, offering better efficiency.
Q: Can you explain how the polling mechanism works in Linux?
A: Linux’s polling lets programs keep an eye on several files for any changes. It uses the poll function. This function checks files, waiting for something to happen, and helps manage I/O smoothly.
Q: What are file descriptors in the context of polling?
A: In polling, file descriptors are unique identifiers for open files or sockets. They’re important for tracking which files are active and need attention.
Q: How does the poll call function?
A: The poll call waits for specific events on file descriptors. It lets you specify which files to watch and for what kind of activities, like reading or writing.
Q: What are the main benefits of using polling in Linux?
A: Polling improves how resources are used, cutting down idle CPU time. It’s great for managing lots of files in busy servers or networks, making systems faster and more scalable.
Q: What are common pitfalls developers face when implementing polling?
A: Developers might read poll events wrong, mishandle file descriptors, or manage errors poorly. These issues can slow down the system or cause bugs.
Q: What are some best practices for implementing polling in Linux?
A: A good approach is to deeply understand poll events. Make sure errors are handled well. Use polling when it truly beats other methods. Writing clear, maintainable code is also crucial.