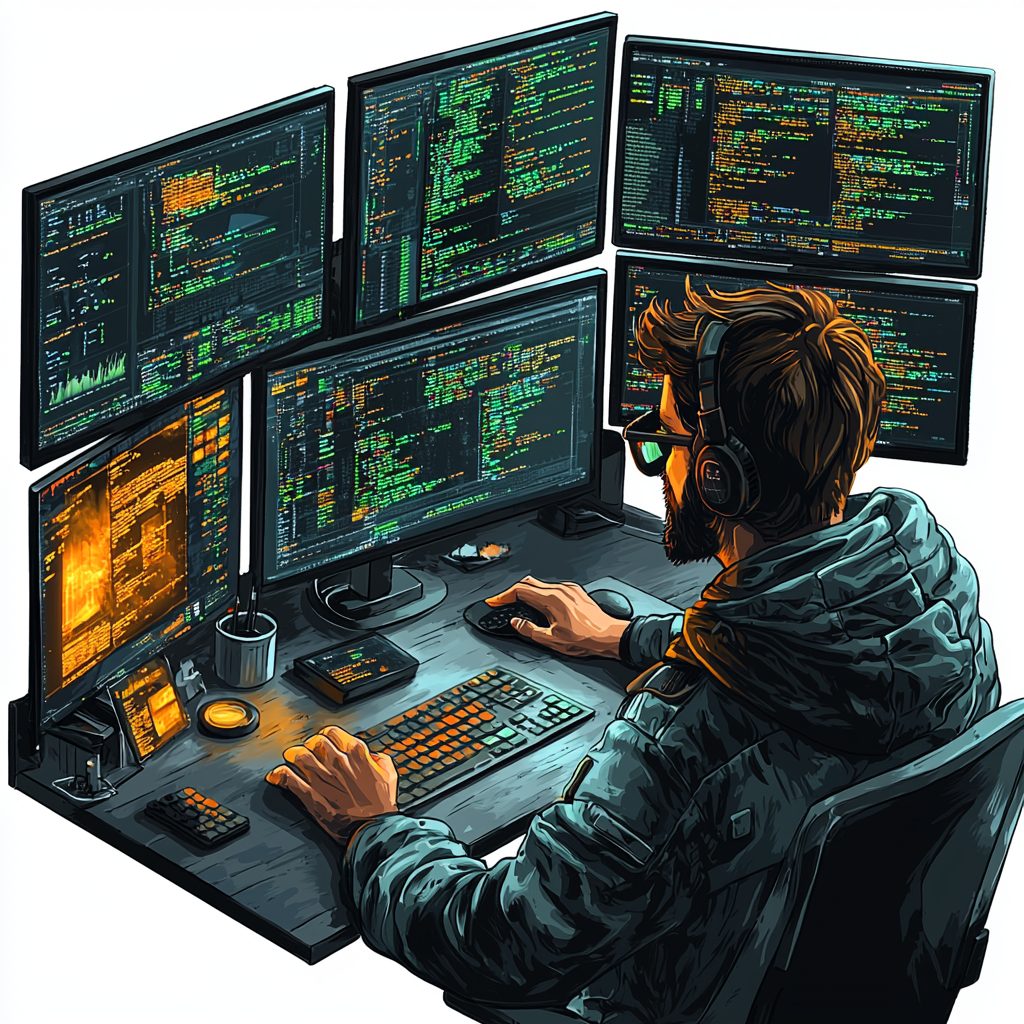
How to Debug a Kernel Module: A Beginner’s Guide
Debugging a kernel module in Linux might sound intimidating at first, but trust me, it’s much more manageable than it seems. Whether you’re new to kernel development or simply curious about how to fix bugs in your module, this guide will walk you through the essential steps, using simple and accessible tools.
We’ll explore various ways to find and resolve issues in kernel modules. From printk to dmesg and gdb, you’ll learn practical debugging methods to make your life easier.
What Is a Kernel Module?
A kernel module is a piece of code that extends the functionality of the Linux kernel. It can be loaded and unloaded dynamically, allowing developers to add drivers or system features without rebooting the machine.
Debugging a kernel module becomes essential when things don’t work as expected, which is a common occurrence in development.
Understanding Why Kernel Debugging Is Different
Unlike user-space programs, debugging kernel modules is a bit tricky. Since kernel code operates in a different space (the kernel space), tools and techniques we use for regular applications don’t always work.
Crashes in the kernel can take the whole system down, so careful debugging is crucial.
Let’s get into some effective debugging techniques!
1. Using printk for Basic Debugging
The simplest and most widely used technique for debugging kernel modules is using printk
. If you’ve worked with printf
in user-space programming, printk
will feel familiar. It prints messages from the kernel to a log, which you can read using dmesg
.
How to Use printk
:
Insert lines of printk
in your module to print messages at key points, like function entry, variable states, or when an error occurs. For example:
printk(KERN_INFO "Loading my kernel module\n");
printk(KERN_DEBUG "Value of x: %d\n", x);
After inserting printk
statements, load your module and check the messages using dmesg
:
dmesg | tail
This will display the latest log entries, including your debug messages.
Tip: Remember to remove or comment out the printk
statements once you’ve finished debugging. Too many log entries can clutter the logs and affect performance.
2. Reading Kernel Logs with dmesg
dmesg
is the kernel message buffer. All messages, including those from printk
, are sent here. Using dmesg
, you can see a detailed list of kernel events, errors, and warnings.
If your module crashes or fails to load, dmesg
often provides the reason.
Run the following command to see the logs:
dmesg | grep mymodule
This filters the logs to show only entries related to your kernel module. It’s a powerful way to identify issues without needing complex debugging setups.
3. Using gdb for Advanced Debugging
For more complex issues, where printk
isn’t enough, gdb
(GNU Debugger) can help. gdb
allows you to step through your code and inspect variables, but kernel debugging with gdb
requires some setup.
To use gdb
for kernel debugging, you’ll need a kernel with debugging symbols enabled. You can then connect gdb
to a running kernel or use gdb
to debug core dumps if the kernel crashes.
Steps to Use gdb
:
- Build your kernel with debugging symbols (
CONFIG_DEBUG_INFO
enabled in the kernel configuration). - Use a remote debugging setup to connect
gdb
to the kernel (this usually involves using QEMU or a remote machine). - Set breakpoints and inspect the flow of your module within the kernel.
While this method is more advanced, it’s invaluable for deep analysis, especially when you need to examine memory or trace complex errors.
4. Crash Dump Analysis
If your module crashes the kernel, you can use tools like kdump
or crash
to analyze the crash dump. This will give you insights into the state of the system when it crashed, helping you pinpoint the issue.
Setting Up kdump:
- Install the
kdump
package on your Linux system. - Enable
kdump
in your system’s settings. - When a kernel crash occurs,
kdump
will capture the crash dump for you to analyze.
With the dump file, you can use the crash
tool to examine the kernel’s state at the time of the crash. This can help identify memory leaks, kernel panics, or other critical issues.
5. Common Pitfalls and Solutions
Here are some common issues you might face when debugging kernel modules, along with solutions:
- Kernel Panic: This usually happens when the module corrupts memory or dereferences a null pointer. Use
printk
orgdb
to trace the flow and identify the problem line. - Module Not Loading: If your module refuses to load, check
dmesg
for error messages. Often, it’s due to mismatched kernel versions or missing symbols. - Memory Leaks: If your module isn’t properly freeing memory, you can use
kmemleak
to detect memory leaks in the kernel.
Conclusion: Debugging Kernel Modules Made Simple
Debugging kernel modules may seem challenging at first, but with the right tools and techniques, it becomes much more manageable.
Whether you’re using printk
for quick and easy checks, dmesg
for logs, or gdb
for more advanced debugging, these methods will help you find and fix issues in your kernel code.
Remember, debugging is an essential part of development. It’s a skill that improves with practice. So, keep experimenting with these tools, and soon enough, you’ll feel confident debugging kernel modules like a pro.
Good luck, and happy debugging!